JVM
JVM이란 Java Virtual Machine의 약자로 자바 프로그램을 실행 시킬수 있는 가상머신이다.
이를 통해 어떤 장치든(PC, 모바일, 서버 등등) 혹은 어떤 운영체제든(Window, linux, MacOs 등등) 자바 프로그램을 실행 할 수 있게 된다.
Java 컴파일러
우리가 작성한 코드(.java파일)을 운영체제가 읽을 수 있게 바이트 코드(.class파일)로 변환해주는 변환기
인터프리터
운영체제가 읽은 바이트 코드를 기기(기계)가 실행할 수 있는 기계어로 번역. JIT(Just In Time) 컴파일러가 인터프리터의 효율을 올려주는 서포터 해석기 역할을 한다.
JDK & JRE
JDK : Java Development Kit
JRE : Java Runtime Environment
JRE는 .class파일을 실행하는 자바 실행 환경이다. JDK는 JRE의 기능 뿐만 아니라 javac 명령어를 통해 .java파일을 .class파일로 변환하고 디버깅 기능까지 포함하고 있다.
변수 & 상수
변수 : 변하는 값
상수 : 변하지 않는 값
// 변수
int byeonSu = 10; // int타입의 byeonSu라는 명을 가진 변수 선언 및 값 할당
byeonSu = 11; // byeonSu의 값을 11로 변경
// 상수
final int sangSu = 10; // int타입의 sangSu라는 명을 가진 상수 선언 및 값 할당
sangSu = 11; // 에러 발생, 상수는 값 변경 불가
변수 타입( 기본형 VS 참조형)
- 기본형 타입(Primitive Type) - 실제 값이 Stack 영역에 저장(메모리 영역에 대해서는 추후에 포스팅)
- 논리형(boolean) : 1byte
- 정수형
- byte : 1byte
- short : 2byte
- int(기본) : 4byte
- long : 8byte
- 실수형
- float : 4byte
- double(기본) : 8byte
- 문자형(char) : 2byte
- 참조형 타입(Reference Type) - 주소값이 Stack영역에 저장. 실제 값은 Heap영역에 저장
- 기본형 타입 8가지를 제외한 나머지
1주차 과제
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
/**
* 입력값
* 1. 요리 제목
* 2. 별점(1~5 사이의 실수 값)
* 3. 요리 레시피 10개의 문장
*/
Scanner scanner = new Scanner(System.in);
// 1. 요리 제목
System.out.print("요리 제목을 입력해주세요 : ");
String recipeTitle = scanner.nextLine();
// 2. 별점
System.out.print("별점을 입력해주세요 : ");
float rate = scanner.nextFloat();
scanner.nextLine();
// 3. 요리 레시피 10 문장
System.out.println("레시피를 입력해주세요 : ");
String recipe1 = scanner.nextLine();
String recipe2 = scanner.nextLine();
String recipe3 = scanner.nextLine();
String recipe4 = scanner.nextLine();
String recipe5 = scanner.nextLine();
String recipe6 = scanner.nextLine();
String recipe7 = scanner.nextLine();
String recipe8 = scanner.nextLine();
String recipe9 = scanner.nextLine();
String recipe10 = scanner.nextLine();
/**
* 출력값
* 1. 대괄호로 요리 제목 감싸줄것
* 2. 별점 소수점 제외한 정수로 표현
* 3. 별점 옆에 퍼센티지 표현
* 4. 레시피 10 문장 앞에 번호 적어줄것
*/
// 1. 요리 제목 대괄호 감싸기
System.out.println("[ " + recipeTitle + " ]");
// 2,3. 별점의 소수점 제외 및 퍼센티지로 나타낼것
int intRate = (int) rate;
double ratePercent = intRate * 100 / 5.0;
System.out.println("별점 : " + intRate + " (" + ratePercent + "%)");
// 4. 레시피 10 문장 앞에 번호 작성
System.out.println("1. " + recipe1);
System.out.println("2. " + recipe2);
System.out.println("3. " + recipe3);
System.out.println("4. " + recipe4);
System.out.println("5. " + recipe5);
System.out.println("6. " + recipe6);
System.out.println("7. " + recipe7);
System.out.println("8. " + recipe8);
System.out.println("9. " + recipe9);
System.out.println("10. " + recipe10);
}
}
< 결과 >
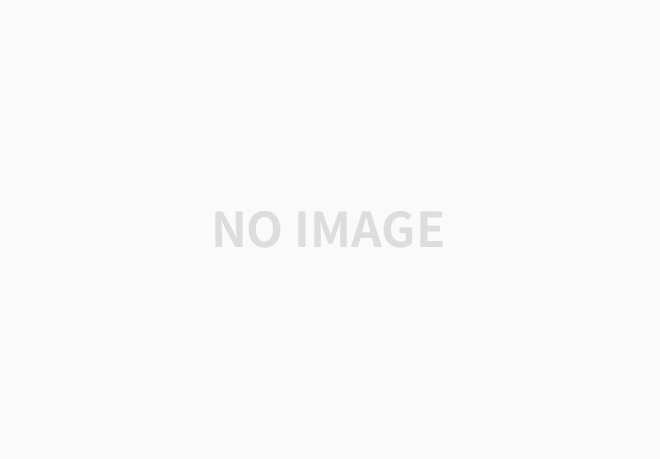
위에 코드에서 nextFloat() 다음에 nextLine()을 하나 추가한 것을 볼수 있다. Scanner에서 nextLine, nextInt, nextFloat 등등 입력을 하고 엔터를 치게되면 입력한 값 맨 끝에 \n도 같이 받게 된다. 그래서 위의 경우 nextFloat() 다음에 nextLine()을 통해 앞에서 받은 \n 개행 문자를 처리해준다.
1주차 EASY~~
'항해99 플러스 > 사전스터디' 카테고리의 다른 글
[TDD방법론 사전스터디] 2주차 (2) | 2024.09.03 |
---|---|
[Java 스터디] 강의 3주차 (1) | 2024.09.03 |
[TDD방법론 사전스터디] 1주차 (4) | 2024.08.26 |
[Java 스터디] 강의 2주차 (0) | 2024.08.21 |
[SpringBoot 사전스터디] 1주차 (0) | 2024.08.19 |